VueJS: Create Multi Root Components
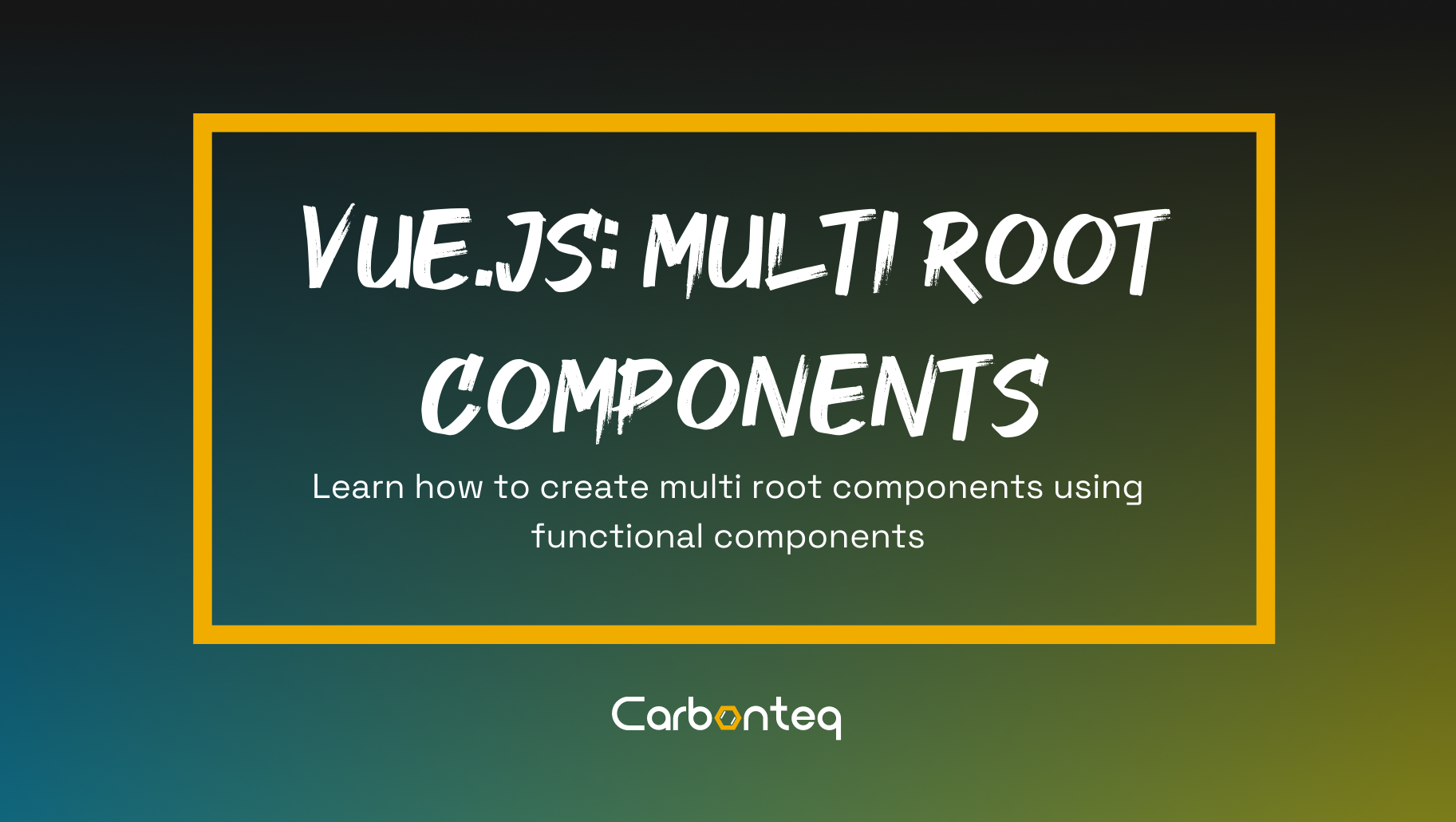
Introduction
Lets face it, during early days of adapting vue js every developer has probably faced this error.
Component template should contain exactly one root element. If you are using v-if on multiple elements, use v-else-if to chain them instead.
and from that point its had coded in our mind that every vue components needs a root element for readers who are new to vue ecosystem.
The right approach
<template>
<div> <!-- The root -->
<p></p>
<p></p>
</div>
</template>
The wrong approach
<template> <!-- No root Element -->
<p></p>
<p></p>
</template>
Multi Root Components
The way around to that problem is using functional components, they are components where you have to pass no reactive data means component will not be watching for any data changes as well as not updating it self when something in parent component changes.
As this is a work around it comes with a price, functional components don't have any lifecycle hooks passed to it, they are instanceless as well you cannot refer to ```this``` anymore and everything is passed with context.
Here is how you can create a simple functional component.
Vue.component('my-component', {
// you must set functional as true
functional: true,
// Props are optional
props: {
// ...
},
// To compensate for the lack of an instance,
// we are now provided a 2nd context argument.
render: function (createElement, context) {
// ...
}
})
Now that we have covered functional components in some detail lets cover how to create multi root components, for that I am gonna present you with a generic example
<template>
<ul>
<NavBarRoutes :routes="persistentNavRoutes"/>
<NavBarRoutes v-if="loggedIn" :routes="loggedInNavRoutes" />
<NavBarRoutes v-else :routes="loggedOutNavRoutes" />
</ul>
</template>
Now if we take a look at NavBarRoutes template
<template>
<li
v-for="route in routes"
:key="route.name"
>
<router-link :to="route">
{{ route.title }}
</router-link>
</li>
</template>
We cant do some thing like this we will be violating single root component restriction
Solution
Make this component functional and use render
{
functional: true,
render(h, { props }) {
return props.routes.map(route =>
<li key={route.name}>
<router-link to={route}>
{route.title}
</router-link>
</li>
)
}
Here you have it you have created a multi root component, Happy coding