Web Animation API
Web animations beautify your website, ease transition, encourage user engagement, and create emotional impact.
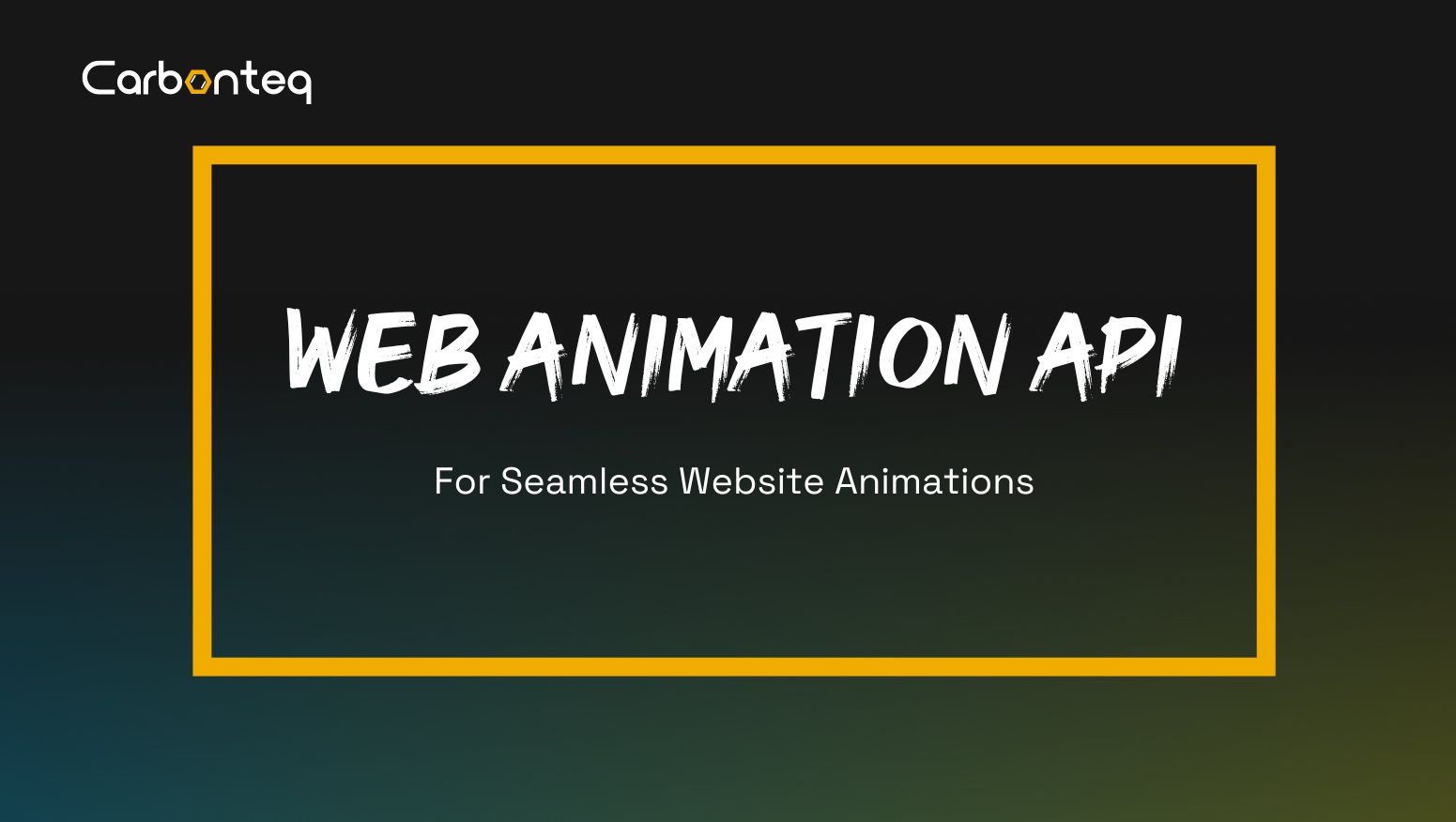
Introduction
Web animations beautify your website, ease transition, encourage user engagement, and create emotional impact. There are different ways to add animations to your website. You can do it using CSS transitions and animations, animated libraries like Framer Motion and GreenStock or you can do it inside your JavaScript. If you follow the latter approach, you will see how much power you have over your animations.
What is Web Animation API (WAAPI)?
WAAPI is a game changer for developers. It is a JavaScript API specifically designed for creating high-performance animations directly in the browser. Its purpose is to provide us with a standardized way to control and manipulate animations on the web, resulting in a smoother and more engaging user experience. It offers more flexibility than traditional CSS animations.
Timing and Animation Models
The timing model in web animations, including the Web Animation API (WAAPI), refers to how time is conceptualized and utilized to control the progression and behavior of animations. The timing model provides a structured way to control the flow of animations over time. It establishes a timeline, defines the duration and delay of animations, specifies the number of repetitions, and incorporates easing functions to create smooth transitions. Understanding the timing model is crucial for orchestrating animations with precision and achieving the desired visual effects.
The animation model in web animations, exemplified by the Web Animation API (WAAPI), centers on the creation and control of animated changes to an element's properties. Keyframes, representing specific states at different points in time, form the foundation. The KeyframeEffect object encapsulates keyframes, timing properties, and the target element, serving as a blueprint for animation. Animation objects, instantiated by associating a KeyframeEffect with an element, act as animation instances. Animation players provide dynamic control over the animation's playback, offering methods to play, pause, reverse, or cancel the animation. This model enables developers to define, customize, and dynamically manipulate animations, fostering a versatile and programmatic approach to web animation development.
const element = document.getElementById('animatedElement');
const keyframes = [
{ transform: 'translateX(0)' },
{ transform: 'translateX(100px)' },
];
const timing = {
duration: 1000, // in milliseconds
iterations: Infinity, // repeat indefinitely
};
const animation = new KeyframeEffect(element, keyframes, timing);
const animationPlayer = new Animation(animation);
animationPlayer.play();
To read more about the two models of WAAPI, click here.
Keyframes
Keyframes are fundamental components of web animations, serving as snapshots that define specific states for an element at distinct moments in time. In the context of the Web Animation API (WAAPI) and other animation frameworks, keyframes are crucial for creating smooth transitions and dynamic visual effects. Keyframes are typically defined as an array of JavaScript objects, where each object represents a specific state of the animated element. These states include properties like position, size, color, or any other style attribute. The browser then interpolates between these keyframes, calculating intermediate states to smoothly transition the element from one keyframe state to another. This interpolation, based on the specified timing model, ensures that animations appear seamless and visually appealing. Keyframes provide a flexible and expressive way to articulate the evolution of an element's appearance over the course of an animation.
const element = document.getElementById('animatedElement');
// Defining keyframes for a simple opacity animation
const keyframes = [
{ opacity: 0 }, // Initial state (completely transparent)
{ opacity: 1 }, // Final state (fully opaque)
];
// Specifying the timing for the animation
const timing = {
duration: 1000, // Animation duration in milliseconds
iterations: Infinity, // Repeat the animation indefinitely
};
// Creating a KeyframeEffect object
const animation = new KeyframeEffect(element, keyframes, timing);
// Creating an Animation object
const animationPlayer = new Animation(animation);
// Playing the animation
animationPlayer.play();
In this example, the element with the ID 'animatedElement' will smoothly transition from being completely transparent (opacity: 0) to fully opaque (opacity: 1) over a duration of 1000 milliseconds. The animation will repeat indefinitely. Click here to learn more about keyframes formats in WAAPI.
Options
In the Web Animation API, the second parameter of the element.animate method is the options object, which allows for fine-tuning and customization of the animation. The options object encompasses various properties, each influencing different aspects of the animation. Here's a brief overview of some key options and their possible values:
- duration (number or string):
- Specifies the duration of the animation in milliseconds or as a string with a time unit (e.g., "2s" for 2 seconds).
- delay (number or string):
- Sets the delay before the animation starts, either in milliseconds or as a string with a time unit.
- iterations (number or string):
- Determines the number of times the animation should repeat. It can be an integer or the string "infinite" for indefinite repetition.
- direction ("normal", "reverse", "alternate", "alternate-reverse"):
- Controls the direction of the animation. "normal" plays the animation forward, "reverse" plays it backward, "alternate" alternates between forward and backward, and "alternate-reverse" starts backward and alternates.
- easing ("linear", "ease", "ease-in", "ease-out", "ease-in-out", cubic-bezier(n, n, n, n)):
- Specifies the timing function for the animation. Predefined values like "ease" and "linear" are available, or you can use a custom cubic bezier function.
- fill ("none", "forwards", "backwards", "both"):
- Determines how the element's style should be styled before and after the animation. "none" (default) leaves the style unchanged, "forwards" keeps the end state, "backwards" applies the start state, and "both" applies both start and end states.
- endDelay (number or string):
- Specifies a delay after the animation has ended, in milliseconds or as a string with a time unit.
- composite ("replace", "add", "accumulate"):
- Defines how the animated value will be combined with other elements. "replace" (default) replaces the value, "add" adds the value, and "accumulate" accumulates values over multiple iterations.
These options provide a versatile set of controls for creating dynamic and customized animations using the Web Animation API. Users can tailor the behavior, timing, and appearance of their animations to suit specific design requirements.
WAAPI vs CSS Animations vs CSS Transitions
CSS Transitions
CSS transitions allow smooth changes in CSS properties from one state to another. For instance, altering an element's background color can be done seamlessly using CSS transitions. They provide control over when the change starts, what properties to animate, and the animation duration.
Properties for CSS Transitions:
- transition-property: Specifies the CSS property to transition.
- transition-duration: Sets the duration of the transition.
- transition-delay: Defines the delay before the animation begins.
- transition-timing-function: Determines the speed of the transition (linear, ease-in, ease-out, etc.).
Shorthand for Transitions:
.element {
transition: <property> <duration> <timing-function> <delay>;
}
Demo:
See CSS transitions in action! In the provided CodePen, hovering on the display changes the background color from black to red.
Multiple Animations:
Demonstrated in the following example, multiple CSS properties can be animated simultaneously using CSS transitions by separating values with commas.
CSS Animations
CSS animations elevate the visual appeal of web elements by allowing intricate animations. Utilizing the @keyframes CSS at-rule, we define rules for the animation's start, end, and intermediary states. Additionally, a rule initializing the CSS animation is essential.
Properties for CSS Animations:
- animation-name: Specifies the name of the @keyframes at-rule.
- animation-play-state: Determines if an animation is running or paused.
- animation-direction: Specifies the animation’s direction.
- animation-iteration-count: Sets the number of animation cycles.
- animation-fill-mode: Dictates how styles apply to the element before and after execution.
Shorthand for Animations:
.element {
animation: <name> <duration> <timing-function> <delay> <iteration-count> <direction> <fill-mode>;
}
Demo: Experience CSS animations in action! In the provided CodePen, witness an object's background color transition from black to red.
Key Differences from CSS Transitions:
- Animations start immediately, not triggered by user interaction.
- The element returns to default styling once the animation concludes.
- CSS animations run both ways by default, requiring explicit definition for reverse functionality.
Multiple Animations: Create diverse animations using CSS animations by grouping properties in a single @keyframes at-rule or using multiple @keyframes at-rules with commas.
WAAPI
The Web Animations API, a JavaScript-based approach to animation, provides developers with enhanced control and subtlety compared to CSS animations. While the initial setup may appear more intricate, the API offers the advantage of separating animation logic from an element's styling, resulting in a more straightforward and easier-to-write solution.
Animating a DOM element with the Web Animations API involves invoking the animate() method on the element. This method takes two parameters: keyframes and options. Similar to CSS transitions and animations, the element must be animatable to utilize this API.
Keyframes
Keyframes in the Web Animations API provide a structured way to define animation states. They can be represented either as an array of keyframe objects or an object with arrays of values.
Example 1: Array of Keyframe Objects:
const keyframesArray = [
{ opacity: 1 },
{ opacity: 0 },
{ opacity: 1 }
];
Example 2: Object with Arrays of Values:
const keyframesObject = {
opacity: [1, 0, 1]
};
Timing Functions and Easing:
Easing, representing the timing function for animations, can be specified for each keyframe individually or for the entire animation.
Example with Individual Easing for Keyframes:
const keyframesWithEasing = [
{ opacity: 1, easing: 'ease-in' },
{ opacity: 0, offset: 0.6, easing: 'ease-out' },
{ opacity: 1, easing: 'ease-in-out' }
];
Example with Easing for Entire Animation:
const keyframesWithOptions = {
opacity: [1, 0, 1],
offset: [0, 0.6],
easing: ['ease-in', 'ease-out', 'ease-in-out']
};
Properties and Methods of the Animation Object:
The animate() method returns an Animation object with various properties and methods.
const animation = element.animate(keyframes, options);
// Example Properties:
const currentTime = animation.currentTime;
const playbackRate = animation.playbackRate;
// Example Methods:
animation.pause();
animation.play();
- currentTime: Represents the current point of the animation in milliseconds.
- playbackRate: Controls the playback speed of the animation.
- Methods like pause(), play(), and reverse() manage the animation's playback.
Demo:
Recreating an animation using the Web Animations API involves utilizing keyframes, options, and methods.
element.animate(keyframes, options);
To play an animation continuously, set iterations to Infinity. For multiple animations, include multiple properties in one keyframes object or create distinct keyframes and options objects.
Mastering these keyframe and options concepts empowers developers to create dynamic and customized animations using the Web Animations API.
Conclusion
Customizing animations on the web becomes seamless with the versatility offered by the properties, methods, and events of the Web Animations API. Through my experience, I find that straightforward animations are often more efficiently executed using CSS, while intricate and complex animations find their strength in JavaScript.
Undoubtedly, creating animations from scratch can pose challenges. For a smoother process, exploring third-party animation tools like Flow is worth considering. These tools can simplify the animation creation process and export animations into formats compatible with the Web Animations API, providing an alternative and efficient approach. Click here to read more about the comparison.
#Pros and Cons of Web Animations
Web animations can add a lot of visual interest and user engagement to your website, but it's important to weigh the pros and cons before diving in.
Pros:
- Enhanced User Experience: Well-crafted animations can guide users through your website, highlight important elements, and create a more interactive and engaging experience.
- Greater Control & Flexibility: The Web Animation API offers more granular control over animation timing, easing functions, and playback compared to CSS animations.
- Complex Animations Made Easy: For intricate animations involving multiple elements or interactions, the Web Animation API simplifies the process compared to complex CSS code.
Cons:
- Performance Issues: Overuse of animations or poorly optimized code can lead to slower loading times and a negative user experience, especially on slower connections.
- Increased Development Time: Implementing animations with the Web Animation API can be more time-consuming compared to simpler CSS animations.
- Browser Compatibility: While widely supported, there might be slight differences in animation rendering across different browsers. Thorough testing is crucial.
When to Use Web Animation API vs. CSS Animations
Use the Web Animation API when:
- You need complex animations with precise control over timing, easing, and playback.
- You're animating multiple elements that interact with each other.
- You want fine-grained control over animation states (play, pause, reverse, etc.)
- Your animation involves interactivity with user input (e.g., hover effects).
Use CSS Animations when:
- You need simpler animations like fades, slides, or rotations.
- Faster development time is a priority.
- Browser compatibility is a major concern (CSS animations are generally more widely supported).
- The animation doesn't require complex interactions or state control.
Libraries built using WAAPI
Other Animation Libraries
- LottieFiles (no-code)
- Animate.css
- AnimeJS
- Popmotion
- Mo.js
- Greenstock Animation Platform (GSAP)
- Velocity.js
- CSShake
- Hover.css
No-code Web Animation Tools
- vev
- jitter
- spline
- webflow
- tumult
- Want to know more about no-code animation tools and how you can collaborate them with Vev? Read this article.
Read this blog by Daniel Wilson to learn more about WAAPI.
If you liked our ideas, click on the subscribe button below to get our latest blogs delivered directly to your email.