What is Koa? A Brief Overview.
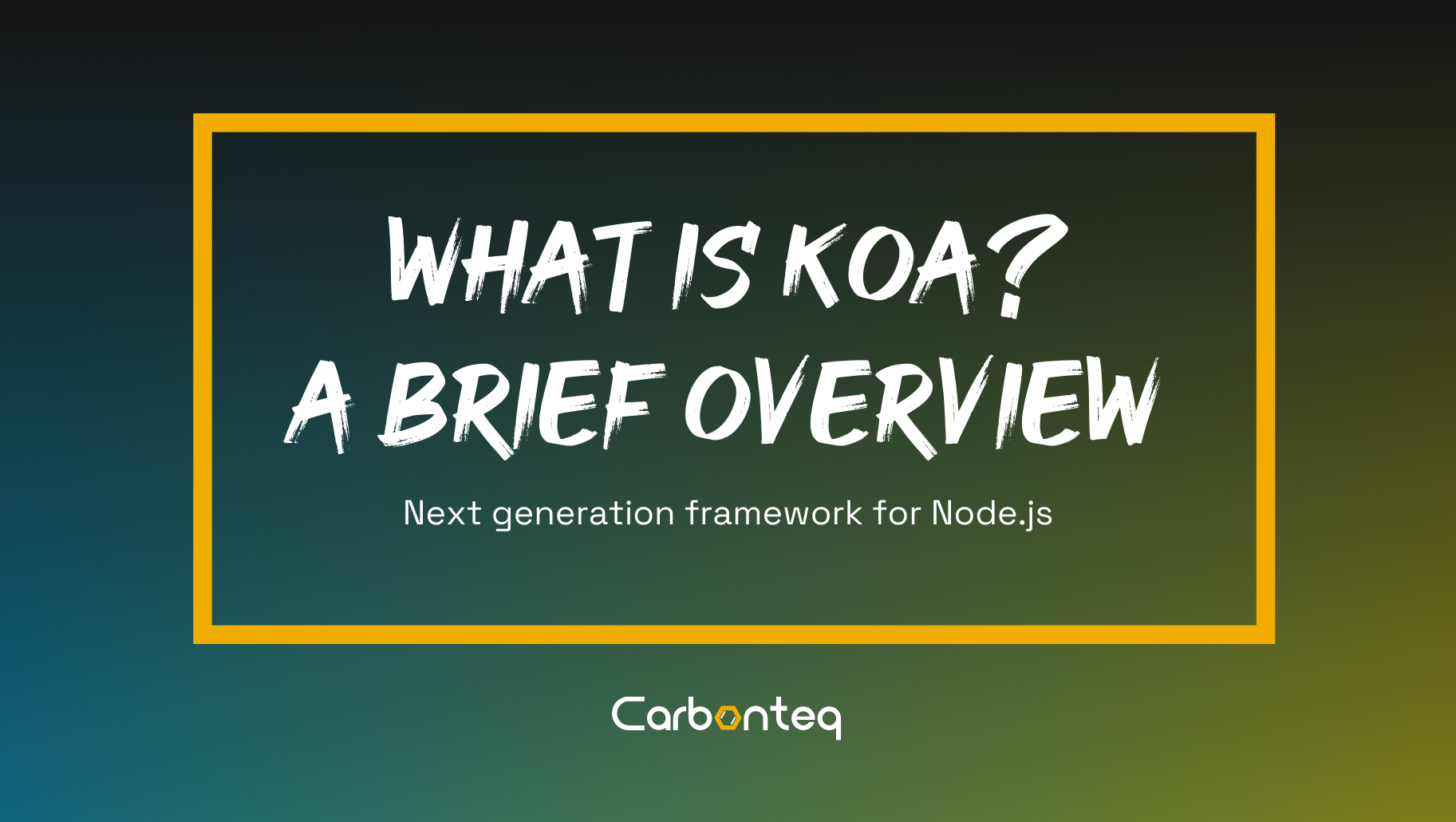
According to official “koa” documentation, koa is:
“Next-generation framework for Node.js”
Koa is created by the team who created the Express Framework. The main reasons for creating koa after Express was the need for a more expressive and robust foundation for APIs and web applications.
Koa uses async functions that mitigates the need for callbacks. This increases the error-handling ability of koa framework.
Origin of Koa
Koa framework takes off where Express.js left. It is accurate to say that Koa is an evolved version of Express. It shares the philosophies of Express and builds on top of them.
The evolution of koa went on at a granular level. It way its code evolved allows us to bypass the older models of working with JavaScript which is the backend of software stack. This ultimately means that there is a streamlined middleware and thus an efficient application.
Middlewear
Middleware substantially improves the performance of an application but exactly what is middleware? Let’s take a look at this definition:
“Any software between the application and the network that encompasses a lot. Think of middleware as plumbing connections for your site—it pipes any communication, like responses and requests, to and fro your application and database/server”
Express, which is the predecessor of koa, for Express JS Middleware it was entirely reliant on “callback”. Koa replaces “callback” with “generators” and repurposed them with a function called “promise” It gives koa the middleware asynchronous capabilities without the intricacies of coding callbacks.
Advantages Of Middleware include:
- No more callbacks.
- Component-based building blocks.
- Cascading Middleware
- Better stream handling
- Better error handling
Koa vs Express
When it comes to rivalry Koa and Express, both have their unique way of doing things. The most differentiating aspects of Koa and Express on which the rivalry is formed are mentioned below:
Koa
- Do one thing well
- Replace
- Async/Await
Express
- Jack of all trades
- REST routes are made simple
- A middleware system
- Parsing the body of POST requests
- Cross site scripting prevention tools
- Automatic HTTP header handling
- Extend
- Callback
Differentiation Between Koa And Other Frameworks
Following are some of the major features that differentiate Koa from other frameworks:
Generators
Koa uses generators according to ECMAScript specifications. These generators help in the upstream and downstream of flow of controls. They can also be used to stop and resume code execution in development stacks. Thus these generators prove to be a prized methods of improving performance of a web application.
Error Handling
With the help of middleware Koa simplifies error handling and makes the process more effective. For custom error-handling such as a centralized login it even add an event listener:
app.on('error', err => {
log.error('server error', err)
});
Cascading Middleware
Normally in Node.js developers need to write additional code in order to stream a file and for closing the stream. Koa solves this by passing the file instead of streaming it. The Yield Next command can be used to yield request downstream and then send back the response upstream.
const Koa = require('koa');
const app = new Koa();
// logger
app.use(async (ctx, next) => {
await next();
const rt = ctx.response.get('X-Response-Time');
console.log(`${ctx.method} ${ctx.url} - ${rt}`);
});
// x-response-time
app.use(async (ctx, next) => {
const start = Date.now();
await next();
const ms = Date.now() - start;
ctx.set('X-Response-Time', `${ms}ms`);
});
// response
app.use(async ctx => {
ctx.body = 'Hello World';
});
app.listen(3000);
Router Module
Koa framework does not support routes. Koa has its own router module which can be used to create routes for website resources like scripts, images, HTML, pages and others. The router module for Koa can be installed with a simple command.
npm install koa-router
HTTP Methods
Operations required by the clients such as GET, PUT, DELETE, POST are understood by the Koa through HTTP methods. It is important to ensure that each coming request from the client includes clear HTTP methods.
Request Object
The request object of Koa is developed on the top of Vanilla response which is provided in Node.js. In Koa it offers many other functionalities. Developer can use the response in Koa to analyse the properties of a response including path, header, URL and even method.
Response Object
Similar to request object, response object is also built upon the vanilla response from Node.js. It also provides similar additional functions as the request object does.
Context
Koa allows developers to encapsulate requests and responses into a single object using Context. This allows developers to create APIs and applications efficiently by using helpful accessors and methods.
A Context is created per request, and is referenced in middleware as the receiver, or the ctx identifier, as shown in the following snippet:
app.use(async ctx => {
ctx; // is the Context
ctx.request; // is a Koa Request
ctx.response; // is a Koa Response
});
A few drawbacks of KOA
There are only a few drawbacks when it comes to using Koa. The most prominent one being that Koa does not have a substantially large following or developer community behind it. It is also not compatible with the middleware of Express Framework. Koa Framework also uses generators which are not compatible with any other Node.js Framework.