Webpack with Code Splitting
In this article, we'll discuss about code splitting. This feature can split code into various bundles after which can be loaded on demand or in parallel.
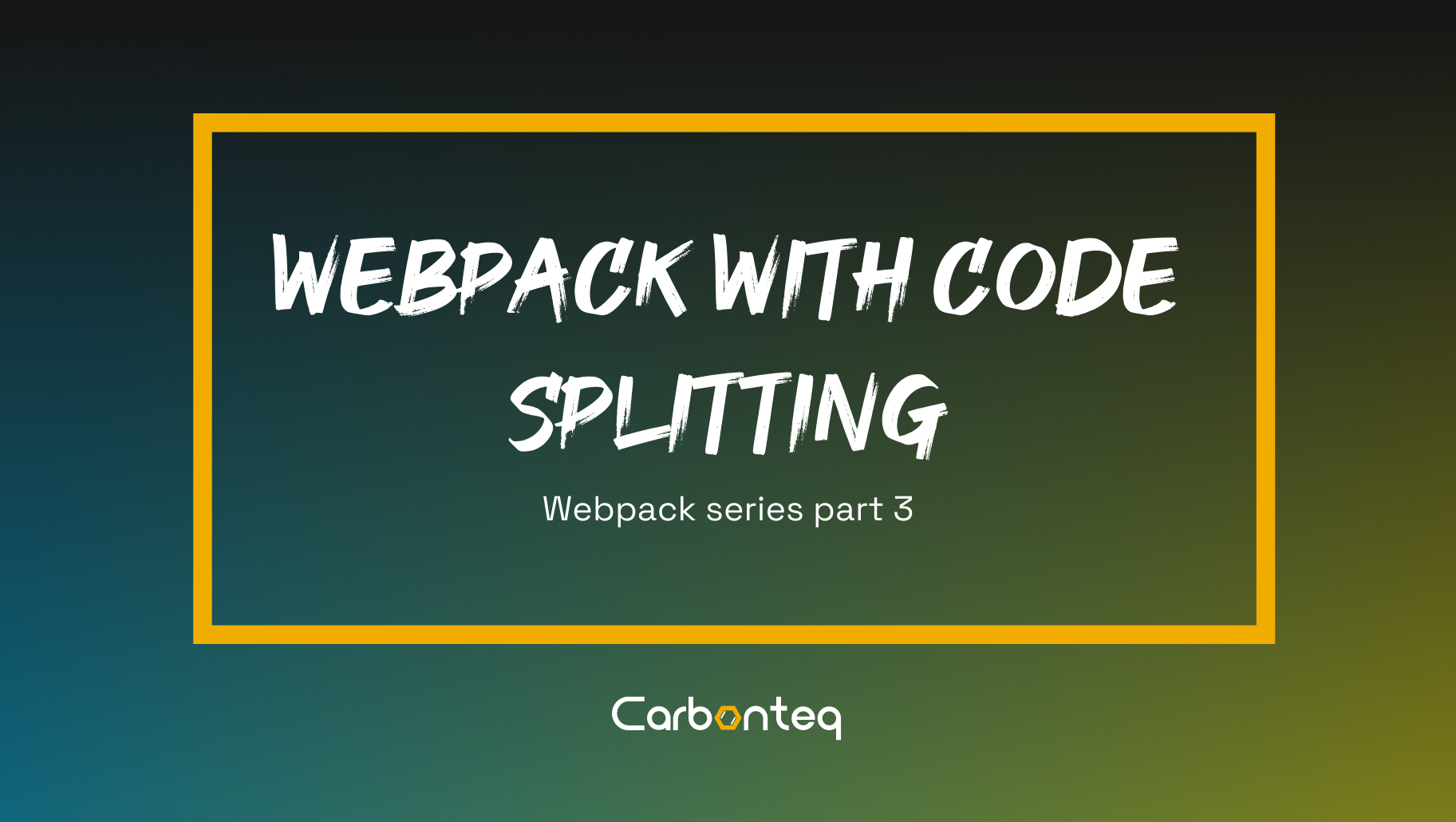
Performance Increase:
The performance on the web is important. Applications and websites should load fast in order to keep the users attention and deliver them a positive experience.
In this article, we will discuss about code splitting.
Code splitting is one of the most riveting features of webpack. By using this feature you can split the code into various bundles after which can be loaded on demand or in parallel. This feature can be used to achieve smaller bundles and control resource load prioritization which, if used correctly, can have a major impact on load time.
For now there are three approaches available for code splitting:
- Entry Points: Manually split code using entry configuration.
- Prevent Duplication: Use the SplitChunksPlugin to dedupe and split chunks.
- Dynamic Imports: Split code via inline function calls within modules.
Entry Points:
This is by far the most easiest way to split code. It is more manual and has some pitfalls we will go over.
const VENDOR_LIBS = ["react","react-dom"]
entry:{
app:'./src/index.js',
vendor:VENDOR_LIBS
}
Prevent Duplication:
The SplitChunksPlugin allows us to extract common dependencies into an existing entry chunk or an entirely new chunk.
Here is the code for SplitChunksPlugin
optimization:{
splitChunks:{
chunks:"all"
}
}
Code Splitting
Code splitting is the most compelling features of webpack.This feature allows you to split your code into different bundles which can be loaded on demand.
Lazy Loading:
One great feature of the web is that we don’t have to make our visitors download the entire app before they can use it. You can think of code splitting as incrementally downloading the app. To accomplish this we’ll use @babel/plugin-syntax-dynamic-import and loadable-component
Webpack has built-in support for dynamic imports, however if you are using Babel then you will need to use the @babel/plugin-syntax-dynamic-import plugin.
npm install @babel/plugin-syntax-dynamic-import --save-dev
So insert the below line into your .babelrc file:
{
"plugins": ["@babel/plugin-syntax-dynamic-import"]
}
Loadable-components is a library for loading components with dynamic imports. It handles all sorts of edges cases automatically and makes code splitting simple
npm install @loadable/component
So first of all we add another directory
mkdir src/components && cd src/components
Now let’s create info.js file into component directory
touch info.js
Then insert below code into info.js file
import React from "react";
const Info = () => <h1>Lazy Loading Component</h1>;
export default Info;
And then import info.js file into your app.js file
So your App.js file look like:
import React from "react";
import loadable from "@loadable/component";
const Info = loadable(() => import("./components/info"));
const App = () => {
return (
<div>
<h1>Hello ReactJs</h1>
<div>
<Info />
</div>
</div>
);
};
export default App;
You must see our previous articles on webpack
Get up and running with webpack and react
Webpack with Hot Module Replacement